Rapid application development using the Opera Unite Yusef library
24th April 2012: Please note
Starting with Opera 12, Opera Unite will be turned off for new users and completely removed in a later release. If you're interested in building addons for Opera, we recommend going with our extensions platform — check out our extensions documentation to get started.
Introduction
Yusef is a framework for Opera Unite applications that provides ready-made solutions to problems such as form validation, access control, UI templating, and more. A lot of functionality is available through Yusef itself, with even more features made available through Yusef plugins.
In a previous article we introduced you to the Yusef library and its plugins. This article will go a little further, looking at a simple application that uses the new version of Yusef provided along with Opera 10.10 (and subsequent releases) to rapidly create applications with a unified UI template (using the UI plugin) and built-in access control (using the ACL plugin). It also demonstrates how to use the new getData()
function to get access to information on the state of the application and its users. The sample application looks like Figure 1.
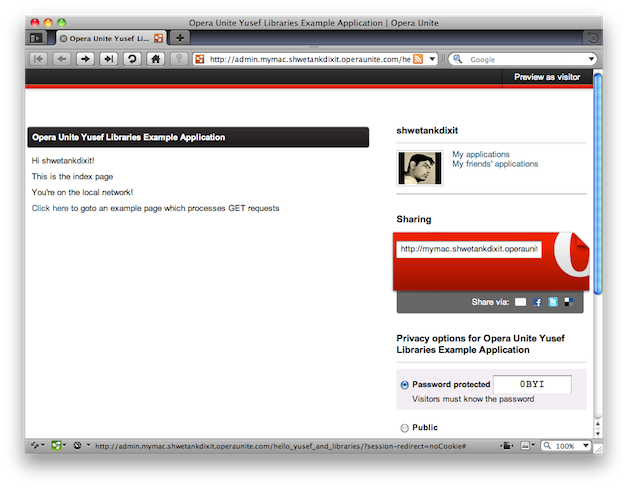
Figure 1: Our sample application.
It’s a good idea at this point to download the sample Opera Unite application so you can follow along with the code.
An updated Yusef
Upon the release of Opera 10.10 final, we made a new version of Yusef available. You can check out the new Yusef by going to the libraries/yusef
folder of the sample Opera Unite application linked above. This new Yusef contains some changes — to start with it makes it easier to retrieve important information about the user and the application. You do this by calling Yusef.getData()
— here is an example of how it can be used:
var data = Yusef.getData(connection);
var username = data.username; // Gets the username of the person if he's logged in
var isOwner = data.visitor.isOwner; // Tells us if the person is the owner of the application or not
var isLocal = data.visitor.isLocal // Tells us if the user is accessing the application through the local network or not
Yusef.getData()
can also give us other useful information such as the origin URL, the application name, the device name, whether the user has cookies, and more.
The ACL and UI plugins
The ACL Yusef plugin is used to restrict access to all or part of your application. The UI Yusef plugin gives your users access to a particular UI template as a default skin, which is stored in the default
subfolder. You can add your own custom skins and switch to them later if you want as well. Using these two plugins will be covered in much more detail in later articles, but for now we’ll just load them as includes in our application using Yusef’s automated library loader, which we will cover below.
The “libraries” package
The example application’s libraries
directory contains a package of essential Opera Unite JavaScript libraries containing many useful functions, for example libraryLoader
, which loads necessary includes into the application, a JSON parsing library, the PSO library, which gives better error messages to the console, and more. You’ll also notice that the directory includes Markuper, Yusef and its plugins.
libraryLoader
We will use Yusef’s automated libraryLoader
to load the necessary plugins — you will see the following code at the top of the index.html
file:
<!DOCTYPE html>
<script src="libraries/libraryLoader/librariesLoader.js"></script>
<script>
Libraries.setTail( 'yusef.translation', 'yusef.ui', 'yusef.acl' );
Libraries.load();
</script>
Here we are including librariesLoader.js
and using its simple syntax to select the plugins we want to use and load them into the application. ui
is used to apply a UI template to the application, acl
controls access to the application by users, and we have also included the translation
library, which provides a way to include translations of the application into different languages.
Server-side JavaScript
All the server-side JavaScript for your Opera Unite applications should reside in the serverScripts
directory — check out the main.js
file contained inside this directory in the example application.
Adding Section Listeners
The next thing you’ll see in main.js
is a section listener that will listen to _index
, and call a function indexFunction
when the index.html
page is called. To make sure that the section loads with the UI template applied, we have to use {ui:true}
as the third argument in the section listener — this calls the UI template to load. The JS code for this looks like so:
Yusef.addSectionListener("_index", indexFunction, {ui:true});
The above code adds a section listener for the index page, which calls the indexFunction()
function, and grants permission for the UI template to be loaded for that function.
Retrieving data
Next we use Yusef.getData()
to retrieve data about access control, UI, etc. and store it in a variable:
function indexFunction(connection) {
/* Get Yusef data (access control, common UI, etc) */
var data = Yusef.getData( connection );
/* Get the application template */
var tmpl = new Markuper( 'templates/hello.html', data );
/* Set data for the application template */
data.content = {
message: "This is the index page"
}
tmpl.parse( data );
return {
title : connection.request.path,
content : tmpl.select( '#hello' ),
styles : [{href: 'styles/style.css'}]
};
}
The Yusef.getData()
function retrieves all the data associated with the UI plugin and the application itself and stores it in the data
variable. The getData()
function returns data about the current access level of the user (from ACL), the UI template they are using, the current application they are using and the type of connection they are using to access the application.
The Markuper
line in the code above calls the HTML template for the application — hello.html
— using Markuper and stores it in the tmpl
variable. You can find the corresponding HTML file in the templates
directory.
Writing the HTML
The main code inside hello.html
looks like so:
<div id="hello">
<span class="roundedBreadcrumbs" />
<h2 class="breadcrumbs"><a href="#">Hello Yusef and Libraries!</a></h2>
<p data-keep-if="visitor.isOwner">Hi {{username}}</p>
<p data-remove-if="visitor.isOwner">Hi Visitor!</p>
<p>{{content.message}}</p>
<p data-keep-if="visitor.isLocal">You're on the local network!</p>
</div>
Remember our data
variable from above, which contains the info from Yusef.getData()
? Since the template is processing all the information from data
, and Yusef.getData()
can provide us with information about the current users of the application, we can use that information to give different content to different users depending on whether they are the owner of the application or just a visitor (on a local network or otherwise).
Handling requests
Any normal web application will probably use GET or POST requests in some form or another. In Opera Unite you can handle them using event.connection.request.queryItems[]
for GET requests and event.connection.request.bodyItems[]
for POST requests. In this article we will just look at handling GET in Opera Unite; POST will follow in a future article.
main.js
contains a section listener that processes the GET input and produces some output:
Yusef.addSectionListener("testingget", theGetFunction, {ui:true});
This makes sure that whenever we go to ourapplication/testingget/
the function theGetFunction()
is called, which processes the results and displays them in another file called another.html
. Let’s look at that function:
function theGetFunction(connection){
var data = Yusef.getData(connection);
var therequest = event.connection.request.queryItems['doesit'][0];
var tmpl = new Markuper('templates/another.html', data);
data.content = {
message: "Hello there!",
message2: therequest
}
tmpl.parse( data );
return {
title : connection.request.path,
content : tmpl.select( '#hello' ),
styles : [{href: 'styles/style.css'}]
};
}
Going back to hello.html
briefly, take note of the following line, which includes the GET call:
<p> <a href="testingget?doesit=works">Click here</a> to go to an example page which processes GET requests</p>
another.html
Now for the another.html
file mentioned above, which displays the data outputted by the theGetFunction
function.
<div id="hello">
<span class="roundedBreadcrumbs" />
<h2 class="breadcrumbs"><a href="#">Hello Yusef and Libraries!</a></h2>
<p data-keep-if="visitor.isOwner">Hi {{username}}</p>
<p data-remove-if="visitor.isOwner">Hi Visitor!</p>
<p>{{content.message}}, the GET request: {{content.message2}}</p>
</div>
Looking at this from top to bottom, the first two lines inside the div
are used to display the standard Opera Unite application rounded corner black box. The next two lines display a custom welcome message depending on whether the person accessing the application is the application owner or not. Finally, the last line displays the data sent via GET.
Conclusion
The libraries we have made available can be used to create Opera Unite applications very quickly and easily. You don't even need to deal with the coding required to make the UI uniform, handle 404 errors, or create an access control system. The libraries can handle all this for you, allowing you to focus on the functionality of the application itself.
This article is licensed under a Creative Commons Attribution, Non Commercial - Share Alike 2.5 license.
Comments
The forum archive of this article is still available on My Opera.
fbc-5be
Friday, November 25, 2011