Building your first Link API application
Introduction
In our Introducing the Opera Link API article, we saw that the Link API works in a RESTful manner, and looked at what you can do with it. This gives you enough information to start working the Link API into your own applications, but to make it easier we have developed libraries to help you get up and running quickly, and a couple of example applications to give you inspiration to write your own.
The libraries provide a high level interface to the API functionality, simplifying the authorization process and data access and manipulation. For now, you have a Python library and a Java library with two examples, a simple Xmarks importer written in Python and a simple Android application to access your notes. Be sure to check Opera's GitHub account for future updates. If you have a GitHub account, you can automatically follow all updates by clicking the "Follow" button on the top right.
This article will show you how to write an application from the ground up using the Python library. To follow this tutorial, you will need a working Python installation, a copy of the pyoperalink library and a recent oauth2 package installed.
Getting consumer keys
For your application to get access to the data, it needs a consumer key (application keys in OAuth parlance) so OAuth can identify it. To get an application key, go to the application registration in the OAuth website (see Figure 1). You will need to login with your Opera account (that's a My Opera, Link, Unite, Portal, widgets.opera.com or dev.opera.com account: they're all the same). If you don't have one, you can go to the My Opera sign up page and get one.
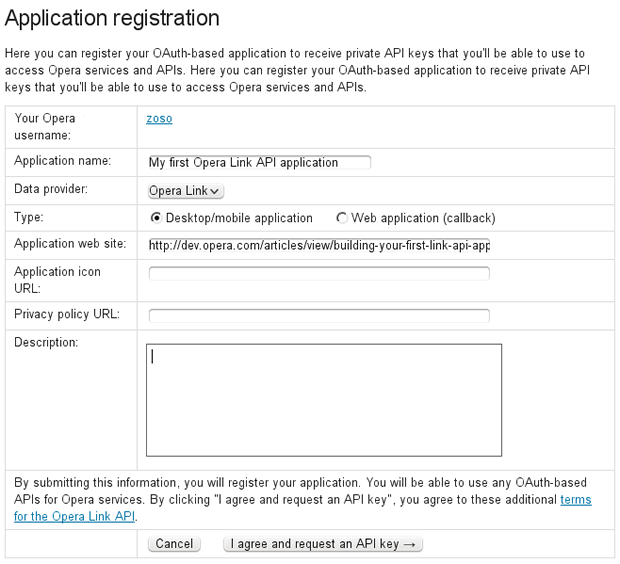
Figure 1: Registering a new OAuth application.
In the application registration page you will have to provide:
- An application name
- An application type (make sure you specify "Desktop/mobile" for this example)
- An application website URL (as this application is just an example and you don't have a website for it, you can give the URL for this article)
- A description
After you click "I agree and request an API key" you will be shown the "Consumer key" and "Consumer secret" (see Figure 2). If you need them again, you can check them by going to your registered applications.
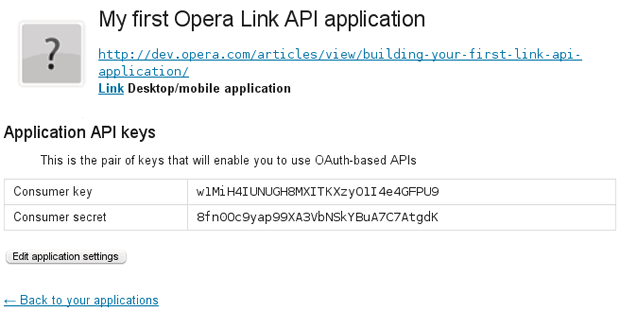
Figure 2: Consumer key and secret for the new application.
Now that you have your application key, you're ready to rock!
Authenticating from Python
Create a new file called duplicateremover.py with the following content, replacing YOUR CONSUMER KEY and YOUR CONSUMER SECRET with the values you got in the previous step):
from pyoperalink.auth import OAuth
from pyoperalink.client import LinkClient
from pyoperalink.datatypes import Bookmark, BookmarkFolder, SpeedDial
consumer_key = 'YOUR CONSUMER KEY'
consumer_secret = 'YOUR CONSUMER SECRET'
auth = OAuth(consumer_key, consumer_secret)
# Get the access token (load from file or get from the server)
try:
with open('access_token.txt', 'r') as token_file:
a_t = token_file.readline().rstrip()
a_t_s = token_file.readline().rstrip()
auth.set_access_token(a_t, a_t_s)
except IOError:
url = auth.get_authorization_url()
print "Now go to:\n\n%s\n\nand type here the verifier code you get:" \
% (url)
verifier = raw_input()
if verifier == "":
print "You need to write the verifier code. Please try again."
sys.exit(1)
token = auth.get_access_token(verifier)
with open('access_token.txt', 'w') as token_file:
token_file.write("%s\n%s\n" % (token.key, token.secret))
The first time you run this application, it will ask you to go to a special URL and type your username and password. Do this and you will see a verifier code that looks like Figure 3:
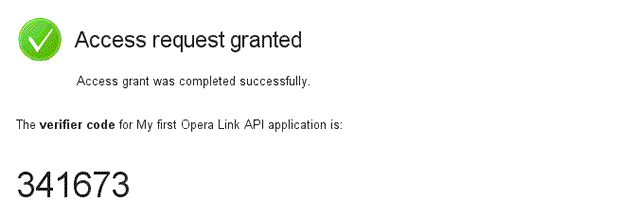
Figure 3: Verifier code from OAuth.
Paste that code into the application and press Enter (see Figure 4). Your application is now authorised to manage the Opera Link data for the account you logged in with.
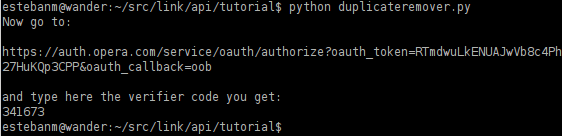
Figure 4: Pasting the verifier code from OAuth into the application.
Accessing some data
Now that we have authenticated, we can start looking at the Opera Link data and manipulating it. In this tutorial we will have a look at the Speed Dial content the account has, and look for bookmarks with the same URL. We will move any such bookmarks to the trash folder.
So let's start by having a look at the current Speed Dial URLs and printing them on to the screen. Add the following text at the end of the duplicateremover.py file:
client = LinkClient(auth)
speeddials = client.get_speeddials()
speeddial_urls = [sd.uri for sd in speeddials]
print "The list of Speed Dial URLs is:", speeddial_urls
The first line there creates an object to access the Link data, and passes the authentication information as a parameter. We then get a list of all dials in your Speed Dial, and extract their URLs in a list by using the property uri. Once we have the list of those URLs, we print them on screen. If you run the example application now, you will see a list of URLs in your terminal.
Inspecting top-level bookmarks
Now let's get the root level bookmarks and see if any of them have the same URLs that appear in the speeddial_urls list. Add the following code to the end of your script:
bookmarks = client.get_bookmarks() # Only top-level bookmarks
for bookmark in bookmarks:
if isinstance(bookmark, Bookmark) and bookmark.uri in speeddial_urls:
print "I should delete the bookmark with title", bookmark.title
Here we are iterating over the top level bookmarks and checking if any of their URLs iare in our list. Notice how we only check the uri property for actual bookmarks, not for bookmark folders or separators, by using isinstance. If when you run this code you don't get anything on your screen, try adding some bookmarks into the root folder pointing to URLs you already have in your Speed Dial (you might want to do that from the Opera Link web interface in My Opera, so your changes are instant).
Removing duplicate bookmarks
Now that you have some bookmarks ready for deletion, modify the code so that the for loop looks like this:
for bookmark in bookmarks:
if isinstance(bookmark, Bookmark) and bookmark.uri in speeddial_urls:
print "Moving bookmark '%s' to Trash" % (bookmark.title,)
client.trash_bookmark(bookmark.id)
If you now run the example again, you will see the familiar Speed Dial URL list, then a line "Moving bookmark '...' to Trash" for every bookmark you already have in your Speed Dial. After running the example, you can go to the web interface in My Opera and check that those bookmarks are now in the Trash folder.
Beyond the basics
So far we have only covered the basics of bookmarks and Speed Dial. For more information, you can read the pyoperalink documentation, the Opera Link API reference documentation, and all the sample code in Opera's GitHub account.
This article is licensed under a Creative Commons Attribution-Noncommercial-Share Alike 3.0 Unported license.
Comments
The forum archive of this article is still available on My Opera.